The Scanner class is placed in java.util package (not in java.io) to facilitate to take input for DS (Data structure) elements. The advantage of Scanner class is parsing is not required for keyboard input data which is required with DataInputStream (as in KeyboardReading1.java) and BufferedReader (as in KeyboardReading2.java).
Scanner class can not only read from keyboard but also is capable to split the input into a number of tokens using the delimiter supplied (default delimiter is whitespace). This is also shown in the next program, KeyboardReading3.java. First and prime advantage of Scanner is it eliminates parsing in keyboard reading.
Following is the class signature of Scanner class as defined in java.util package.
Keyboard Input Scanner Java – No Parsing
The keyboard input is read with nextXXX() methods of Scanner class.
import java.util.Scanner;
public class KeyboardReading3
{
public static void main(String args[])
{
Scanner scan = new Scanner(System.in);
// reads a whole line
System.out.println("Enter your name:");
String s1 = scan.nextLine();
System.out.println("Your name is " + s1);
// reads only one word
System.out.println("City name:");
String s2 = scan.next();
System.out.println("City is " + s2);
// reading an integer
System.out.println("Enter whole number:");
int x = scan.nextInt();
// reading a double
System.out.println("Enter double value:");
double y = scan.nextDouble();
System.out.println("Number are " + x + " and " + y + " and their product is " + (x*y));
// all data entering at a time in a single line
System.out.println("Enter name, age, maraks, height (floating-point) and \npromoted or not (boolean) (giving whitespace):");
String name = scan.next();
byte age = scan.nextByte();
short marks = scan.nextShort();
float height = scan.nextFloat();
boolean promoted = scan.nextBoolean();
System.out.println("The values entered: " + name + ", " + age + ", " + marks + ", " + height + " and " + promoted);
scan.close();
}
}
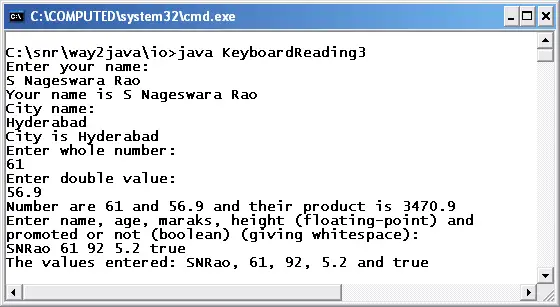
This way of taking keyboard input is much easier as parsing is not required by the programmer. The Scanner class includes special methods like nextLine(), next(), nextByte(), nextShort(), nextFloat() and nextBoolean() etc. which parses to the appropriate data type implicitly. These methods are used in the above program.
The nextLine() method reads whole line that may contain whitespaces in the middle of the input. The next() method reads one word only delimited by whitespace. For example, if the user enters "Hello World", the next() method reads only "Hello" leaving "World".
Similarly methods like nextLong() etc. also exist that can read long data type directly.