Before going into the details of this server-to-client communication, it is advised to go through Networking – Introduction and Communication with TCP/IP Protocol to know the terms and basics of networking and the way Java supports.
Total 4 applications are available on TCP/IP sockets.
Application Number | Functionality |
---|---|
1st application | Client to server communication (one-way) |
2nd application | Server to client communication (one-way) |
3rd application | Server sends file contents to client (two-way, non-continuous) |
4th application | Chat program (two-way, continuous) |
This is the second application (first one being client-to-server) in one-way communication. In one-way communication, either client sends to server or server sends to clients. But one will not reciprocate (reverse communication) with the other. In two-way communication, client sends to server and also server sends back to client.
2nd application Server to client Example Java: Server to client communication
It is just exactly the reverse of the previous client-to-server application. Here, the server sends and client receives. For this reason, now the client uses input streams and the server uses output streams.
The client-side program, DateInfoClient.java receives thanks message from the server along with the current date and the server-side program, DateInfoServer.java sends thanks with date particulars.
Client Program – DateInfoClient.java
Responsibility: To receive the message from the server. As it is receiving, it uses input streams.
import java.io.*;
import java.net.*;
public class DateInfoClient
{
public static void main(String args[]) throws Exception
{
Socket sock = new Socket("127.0.0.1", 7000);
InputStream istream = sock.getInputStream();
BufferedReader br1 = new BufferedReader(new InputStreamReader(istream));
String s1 = br1.readLine();
System.out.println(s1);
br1.close(); istream.close(); sock.close( );
}
}
BufferedReader br1 = new BufferedReader(new InputStreamReader(istream));
In the earlier client-to-server application, we used DataInputStream and in this place the character stream BufferedReader is used. The readLine() method of BufferedReader is not deprecated.
Server Program – DateInfoServer.java
Responsibility: To send a message to client. As it is sending, it uses an output streams.
import java.net.*;
import java.io.*;
public class DateInfoServer
{
public static void main(String args[]) throws Exception
{
ServerSocket sersock = new ServerSocket(7000);
System.out.println("Server ready........");
Socket sock = sersock.accept( );
OutputStream ostream = sock.getOutputStream();
BufferedWriter bw1 = new BufferedWriter(new OutputStreamWriter(ostream));
String s2 = "Thanks client for your calling on " + new java.util.Date();
bw1.write(s2);
bw1.close(); ostream.close(); sock.close(); sersock.close( );
}
}
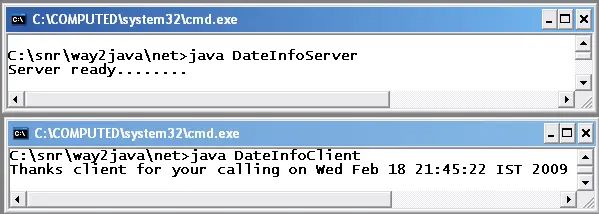
Screenshot on Server to client Example Java
The same terminology, methods and execution steps of the first application are used and requires no explanation again. Now the port number used is 7000. Whether client sends or server sends, always, the server should bind the connection (using ServerSocket) and for this reason run server program first.
Java comes with many classes grouped as categories. A group gives common properties. A few groups are given below.
1. Wrapper classes
2. Listeners
3. Adapters
4. Layout managers
5. Byte streams
6. Character streams
7. Print streams
8. Filter streams
9. Wrapper streams
10. Components
11. Containers
12. Thread groups
13. Exceptions